How to Dynamically Modify Roles Using the Digital Samba SDK
Want to adjust participant roles during a video conference effortlessly? With the Digital Samba SDK, it's easy! This powerful SDK lets you dynamically modify roles, granting or revoking permissions on the fly. Need to mute or unmute, revoke or grant broadcast permission, or manage access to specific features? The Digital Samba SDK gives you the tools for seamless role management within your video conferencing platform. That being said, let's explore how this innovative SDK can help make your meetings better!
Table of Contents- What are roles and permissions?
- Modifying roles with the Digital Samba SDK
- Granting and revoking broadcast permission
- Remote mute and request to unmute
- Allow or disallow screen sharing
- Changing roles
- Checking permission with permissionManager
- Conclusion
What are roles and permissions?
Imagine you're hosting a virtual meeting or webinar. Who controls the microphone? Who can share their screen? Or who can kick out disruptive participants? These questions are all answered by roles and permissions.
Roles and permissions dictate the actions users can take within a video conference. Roles are predefined sets of permissions that determine a user's access level, from complete control as a moderator to limited viewing as an attendee. Defining clear roles and permissions ensures meetings run efficiently.
You can manage roles through Digital Samba’s API, Dashboard, and SDK. Here are some of the permissions you can set in a role from the default roles: Moderator, Speaker, and Attendee:
- Broadcast
- Manage broadcast
- Start/End session
- Remove participants
- Share screen
- Record session
- Chat in general chat
- Remote mic/camera control
- Raise hand
- Edit whiteboard
- Manage edit-whiteboard permission
This granular control provides freedom to create customised video conferencing experiences that meet your users' needs - whether a small team meeting or a large-scale webinar.
Modifying roles with the Digital Samba SDK
To enhance your video conferencing experience, you can dynamically modify roles and permissions using Digital Samba Embedded SDK methods and events. These SDK methods provide developers with the flexibility to create advanced features and behaviours for their video conferencing solutions, improving the overall user experience. With these methods and events, you can programmatically:
- Grant and revoke broadcast permissions
- Remote mute and request to unmute
- Allow or disallow screen share
- Change role
- And lots more!
We’ll learn how to implement all the functionalities above in the following sections.
Prerequisites:
- A Digital Samba developer account (sign up here)
- Have the Digital Samba Embedded SDK integrated into your setup. See the integration guide.
- A computer with internet connectivity
- Basic knowledge of JavaScript/TypeScript
If you have met all the requirements above, you're good to go with the steps below:
1. Granting and revoking broadcast permission
The allowBroadcast() SDK method grants broadcast permissions to a target user, allowing them to share media in webinar scenarios where most participants don't need video/audio capabilities by default. To use this method, call it with the target user's ID as a parameter, like this:
To revoke broadcast permission, call the disallowBroadcast() method with the same user ID:
Note: disallowBroadcast() only takes effect if allowBroadcast() was previously called for that user.
2. Remote mute and request to unmute
The requestMute() method allows you to mute a participant's microphone by passing their user ID to the method. A popup will appear, prompting the initiator to confirm they want to mute the participant.
Example:
This method can be used when a moderator needs to silence a disruptive participant, such as when background noise is caused by someone. The moderator can then mute the participant until the issue is resolved.
To request an unmute, use the requestUnmute() method. This method asks a muted participant to unmute their microphone by passing their user ID. A popup will appear, allowing the participant to confirm or deny the request.
Note: You cannot force an unmute; the participant must agree to it.
sambaFrame.requestUnmute('9efc7798-a1a4-44bd-9d8d-532fe0feea9d');
This method can be used when a moderator has previously muted someone and now wants to let them speak again. The participant will receive a popup to re-enable their microphone.
3. Allow or disallow screen sharing
The allowScreenshare() method grants screen share permissions to a specific user, enabling them to share their screen. The user's ID is provided as a parameter, and they will be able to share their screen after this method is called. Here’s how to use the allowScreenshare() method:
This functionality can be useful in scenarios where particular user roles do not have screen share permissions by default, such as in webinars. It allows for the selective granting of screen share permissions to specific users.
On the other hand, the disallowScreenshare() method revokes the screen share permissions for a specific user, and it only takes effect if allowScreenshare() was previously called for that user.
This method can be used to revoke screen share permissions for particular users, especially in scenarios where these permissions need to be granted and revoked dynamically during a webinar, perhaps by moderators using a custom participants panel in an integration.
4. Changing roles
The changeRole() method is used to modify the role of a user by providing their user ID and the new role as parameters. For example, to promote a user to a moderator and then later demote the moderator to an attendee, the following code can be used:
sambaFrame.changeRole('9efc7798-a1a4-44bd-9d8d-532fe0feea9d', 'attendee');
This method is typically employed in scenarios where a custom participants panel in an integration allows power users to change the roles of other participants. Additionally, the method often triggers an event after a user's role is changed.
The roleChanged event is emitted when a user's role changes in a session. This event can be useful for displaying up-to-date roles in a custom participants panel or for reacting to role changes in other ways. The event payload typically includes the user's ID and the role they have transitioned from and to.
To subscribe to this event, use the on() method and provide a callback function that will handle the event:
const data = event.data;
console.log('User', data.userId, 'role changed from', data.from, 'to', data.to);
});
Here’s a sample payload:
"type": "roleChanged",
"data": {
"userId": "2775f82-845e-4416-82c9-04cc25669c0b",
"from": "attendee",
"to": "moderator"
}
}
}
5. Checking permission with permissionManager
The permissionManager provides access to a module for managing permissions. It includes the hasPermissions() method, which is used to check if the local user has specific permissions. The method takes the permissions to check for and an options object as parameters. The PermissionTypes enum lists the various types of permissions that can be checked:
broadcast = 'broadcast',
manageBroadcast = 'manage_broadcast',
endSession = 'end_session',
startSession = 'start_session',
removeParticipant = 'remove_participant',
screenshare = 'screenshare',
manageScreenshare = 'manage_screenshare',
recording = 'recording',
generalChat = 'general_chat',
remoteMuting = 'remote_muting',
askRemoteUnmute = 'ask_remote_unmute',
raiseHand = 'raise_hand',
manageRoles = 'manage_roles',
}
HasPermissionsOptions {
targetRole?: string;
role?: string;
userId?: UserId;
}
Here's an example of using the hasPermissions() method to check if the local user can broadcast:
console.log('Local user can broadcast:', canBroadcast);
The HasPermissionsOptions object allows for additional options when checking permissions, such as specifying the target role, user role, or user ID.
This can be useful in scenarios where you need to dynamically manage and check permissions, such as in custom participants panels or when implementing role-based access control.
For more information on the Digital Samba Embedded SDK role and permission methods or other methods, please visit the SDK documentation.
Conclusion
The Digital Samba SDK offers seamless role management, allowing dynamic modification of participant roles and permissions during video conferences. This powerful tool empowers users to adjust roles, grant or revoke permissions, and manage access to specific features on the fly. By providing granular control and flexibility, the SDK enables the creation of customised and efficient video conferencing experiences. With its embedded methods and events, developers can enhance the overall user experience, making meetings better and more productive.
Share this
You May Also Like
These Related Stories
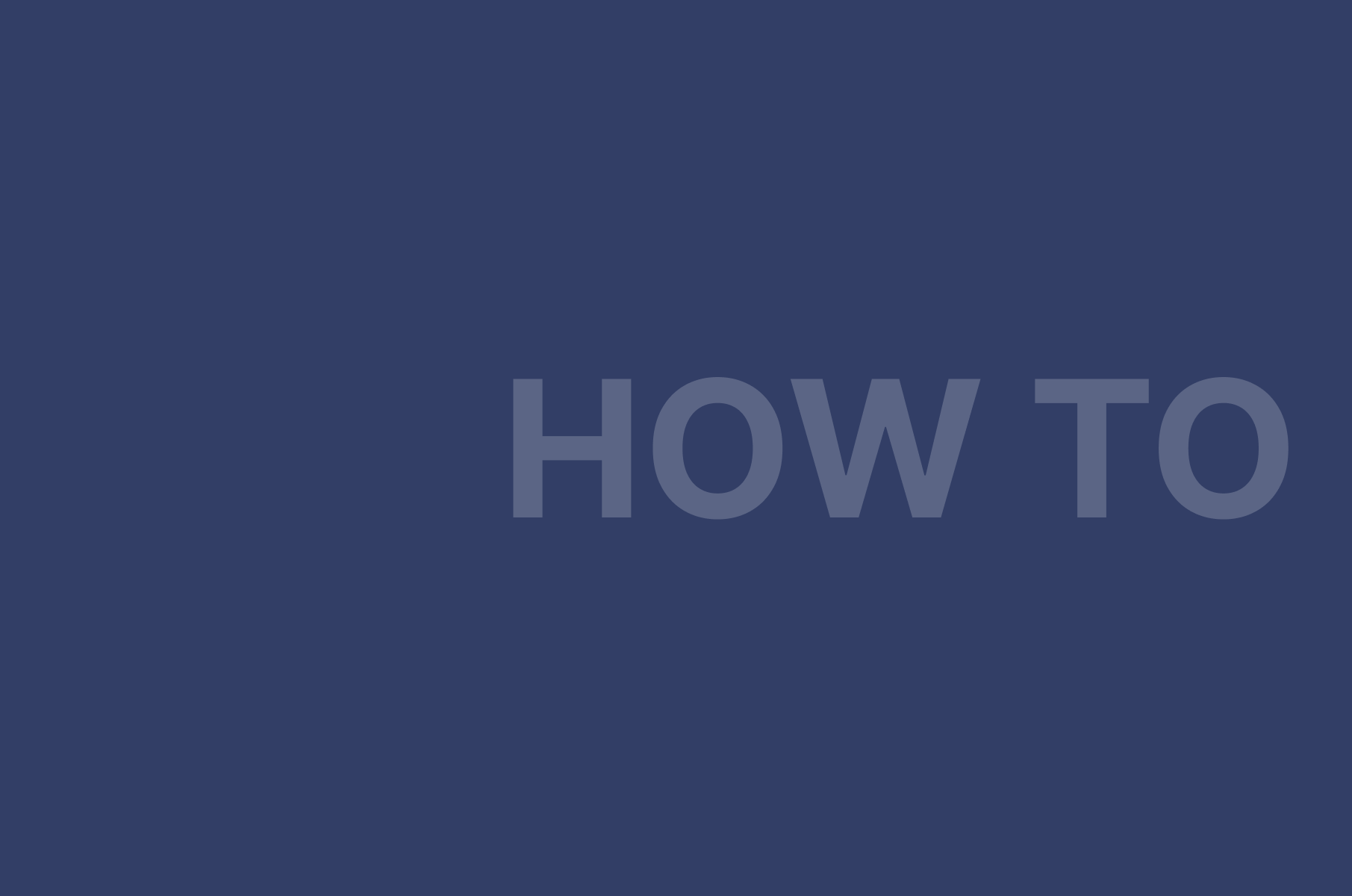
How to Join and Control the Embedded Video Conference with the Digital Samba SDK
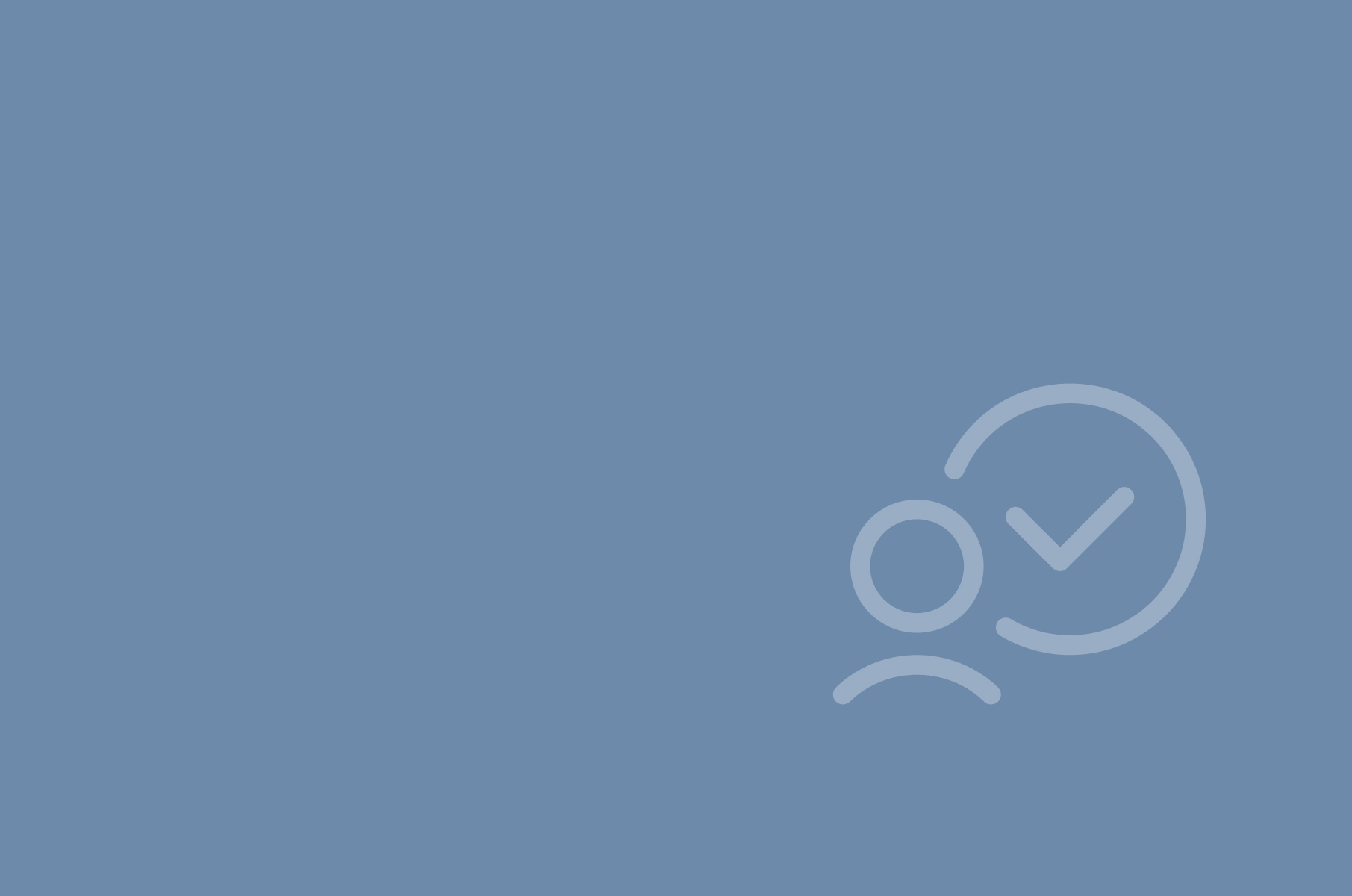
Exciting Update: Introducing Roles and Permissions for Digital Samba's Video Conferencing API & SDK
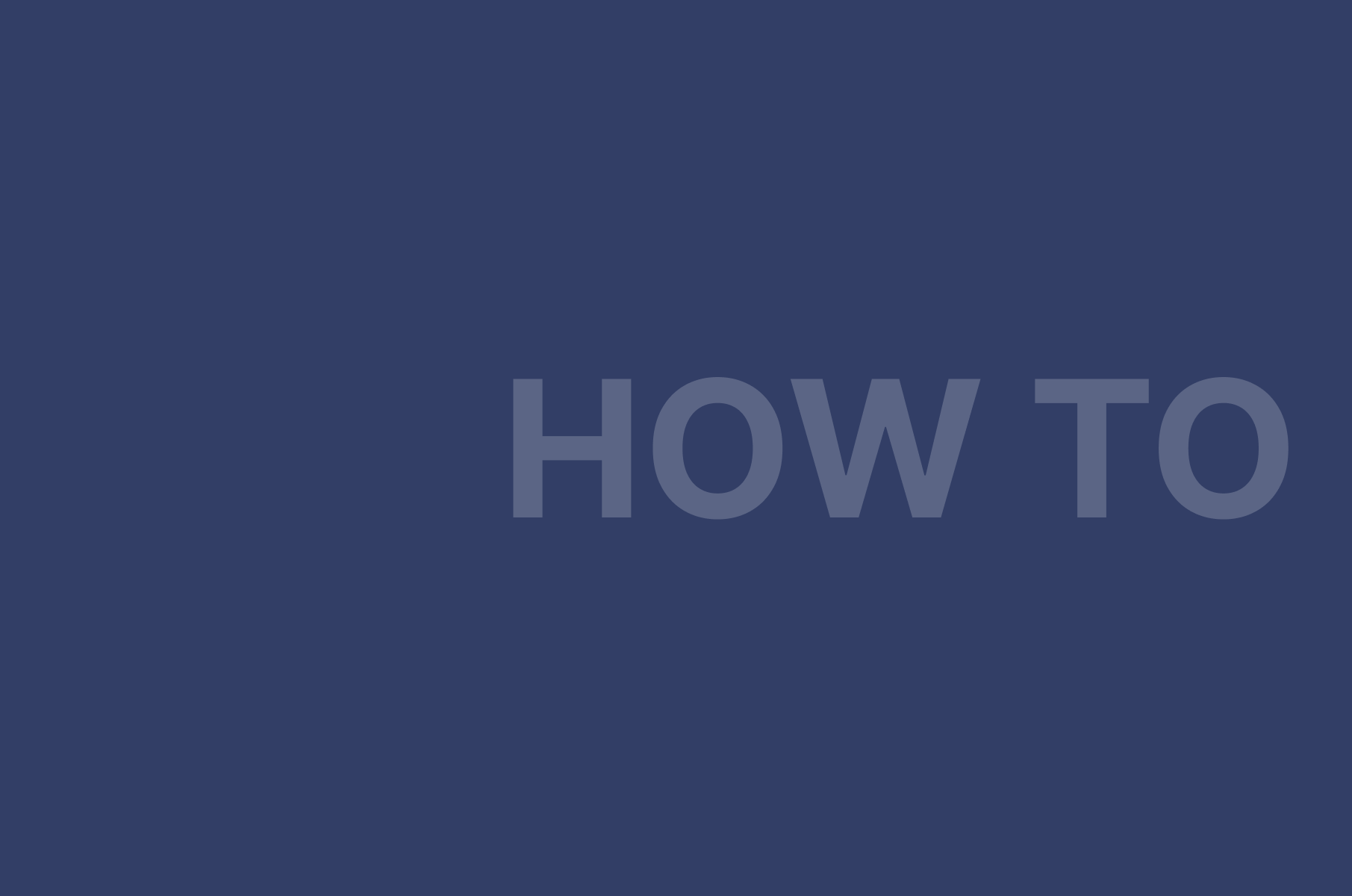