How to Configure Your Room Defaults in Digital Samba Using the Developer API
In the dynamic landscape of virtual communication, Digital Samba has emerged as a prominent solution for seamless video conferencing and online meetings. Leveraging its developer API, you can now take control of your virtual spaces to enhance user experience and tailor settings according to your unique requirements.
In this article, we’ll delve into configuring room defaults in Digital Samba, and shed light on the significance of room inheritance, how to configure specific rooms, and ultimately, empowering users to optimise their virtual interactions.
Table of Contents
- Understanding room settings inheritance in Digital Samba
- How to configure rooms on a per-room basis using the developer API
- How to configure your room defaults using the developer API
- Customise video calls on your web mobile application with the Digital Samba video conferencing API
Understanding room settings inheritance in Digital Samba
Before delving into the specifics of room defaults, it's crucial to grasp the concept of room inheritance.
Digital Samba employs a hierarchical structure, where settings configured at a global scale (default room settings) are automatically inherited by individual rooms unless you configure the individual room specifically. This approach ensures consistency and efficiency by allowing users to define defaults inherited by individual rooms.
So, for instance, if you create a new room and don’t tweak its primary colour or background colour, and then a team admin or moderator changes the primary colour to green in the default settings, your new room’s primary will automatically be altered to green.
Let’s explore how this works when configuring your room using the developer API.
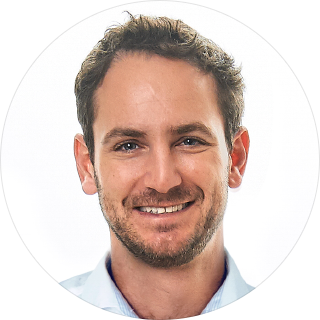
Contact our team today!
Add customisable video chat to your website or application with the Digital Samba API and SDK
How to configure rooms on a per-room basis using the developer API
To harness the full potential of the Digital Samba developer API, you can take a more granular approach to configure your room by customising settings for individual rooms.
You have two options for configuring your Digital Samba room:
Start by making a POST request to the /rooms endpoint. This method is used to set up your room's configuration during its creation. The highlighted section shows the room configurations you set when you create the room
"https://api.digitalsamba.com/api/v1/rooms"
);
const headers = {
"Authorization": "Bearer base64(teamId:teamDeveloperKey)",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"topic": "standup",
"friendly_url": "my-standup",
"privacy": "public",
"external_id": "myExtID123",
"default_role": "moderator",
"roles": [
"moderator"
],
"topbar_enabled": true,
"toolbar_enabled": false,
"toolbar_position": "right",
"toolbar_color": "#FF0000",
…
"primary_color": "#008000",
"background_color": "#000000",
"palette_mode": "light",
"language": "en",
"max_participants": 50
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Alternatively, you can execute a PATCH request to the /rooms/:id endpoint. This approach is useful when you need to modify the settings of an existing room. Simply provide the id of the room you want to edit and the changes will be applied accordingly.
Important: Do not leak your developer key into the client’s browsers. Execute these requests only from your backend (server-side). Note you must do a base64 encoding of the: concatenation of your teamId and developer key, but this is out of the scope of the article. Every major language provides built-in functions to base64 encode data.
"https://api.digitalsamba.com/api/v1/rooms/a853d608-e6cf-48eb-a3c9-7d089bbc09b0"
);
const headers = {
"Authorization": "Bearer base64(teamId:teamDeveloperKey)",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"topic": "standup",
"friendly_url": "my-standup",
"privacy": "public",
"external_id": "myExtID123",
"default_role": "moderator",
"roles": [
"moderator"
],
Fields_you_want_to_edit_go_here,
};
fetch(url, {
method: "PATCH",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Please ensure you have the API credentials for authentication before executing the PATCH request. This involves adding your team_id and developer_key, which you can access in the dashboard’s ‘Team’ option, as shown below.
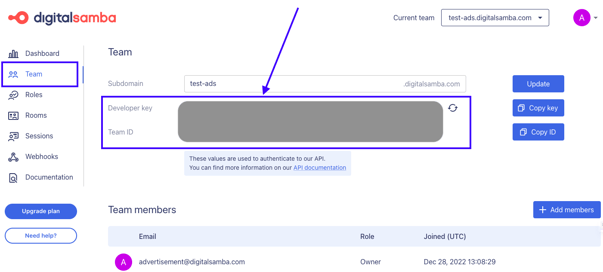
To edit a room, add the fields you want to configure in the data parenthesis.
For example, if you are holding a lecture or training course, turn off screen sharing for attendees. Even if you've enabled screen sharing in your default room settings using the developer API, it’ll be turned off for this specific room. You might also want to enable the chat feature and ensure the UI is in English.
Let’s see how this looks with a PATCH request code example. We will use JavaScript, but Digital Samba allows you to achieve the same with PHP, bash, or Java. Explore more here.
"https://api.digitalsamba.com/api/v1/rooms/a853d608-e6cf-48eb-a3c9-7d089bbc09b0"
);
const headers = {
"Authorization": "Bearer base64(teamId:teamDeveloperKey)",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"topic": "standup",
"friendly_url": "my-standup",
"privacy": "public",
"external_id": "myExtID123",
"default_role": "moderator",
"roles": [
"moderator"
],
"language": "en",
"language_selection_enabled": false,
"chat_enabled": true,
"screenshare_enabled": false
};
fetch(url, {
method: "PATCH",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
The fetch() function will make a request to the API to update that specific room with the new settings included in the PATCH request, as such, updating the room.
How to configure your room defaults using the developer API
While configuring specific rooms provides tailored solutions, room defaults are your foundation for consistency across multiple rooms. Setting defaults saves time and maintains a standardised experience for users.
Unlike configuring a specific room, you must execute a PATCH request against the /api/v1 endpoint, as highlighted below.
"https://api.digitalsamba.com/api/v1"
);
const headers = {
"Authorization": "Bearer base64(teamId:teamDeveloperKey)",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"domain": "aspernatur",
"default_role": "moderator",
"roles": [
"moderator"
],
Fields_you_want_to_edit_go_here
};
fetch(url, {
method: "PATCH",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
For example, suppose your goal is to ensure a uniform brand identity throughout your video conferences. In that case, you can achieve this by implementing the following settings: a top bar, a right-positioned toolbar with a green colour scheme, utilising green as the primary colour, incorporating a white background, prominently displaying your logo, maintaining an English-language user interface, and fortifying security across all rooms through the activation of end-to-end encryption (E2EE).
"https://api.digitalsamba.com/api/v1"
);
const headers = {
"Authorization": "Bearer base64(teamId:teamDeveloperKey)",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"domain": "aspernatur",
"default_role": "moderator",
"roles": [
"moderator"
],
"topbar_enabled": true,
"toolbar_enabled": true,
"toolbar_position": "right",
"toolbar_color": "#3CBO43",
"primary_color": "#3CBB043",
"background_color": "#FFFFFF",
"language": "en",
"full_screen_enabled": false,
"e2ee_enabled": true,
"logo_enabled": true,
"custom_logo": "natus",
};
fetch(url, {
method: "PATCH",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
As mentioned above, the main difference between configuring a specific room and updating your default room settings is the endpoint to which you execute the PATCH request.
With the Digital Samba video conferencing API, you can update as many fields for your default rooms as you wish. See our documentation for a more detailed list of the fields you can configure.
Customise video calls on your web mobile application with the Digital Samba video chat API
Configuring room defaults using the developer API in Digital Samba marks a transformative step towards enhancing virtual interactions. By embracing the concepts of room inheritance and targeted configurations, you can create a virtual environment that aligns seamlessly with their needs.
Get started today with a free account.
Share this
You May Also Like
These Related Stories
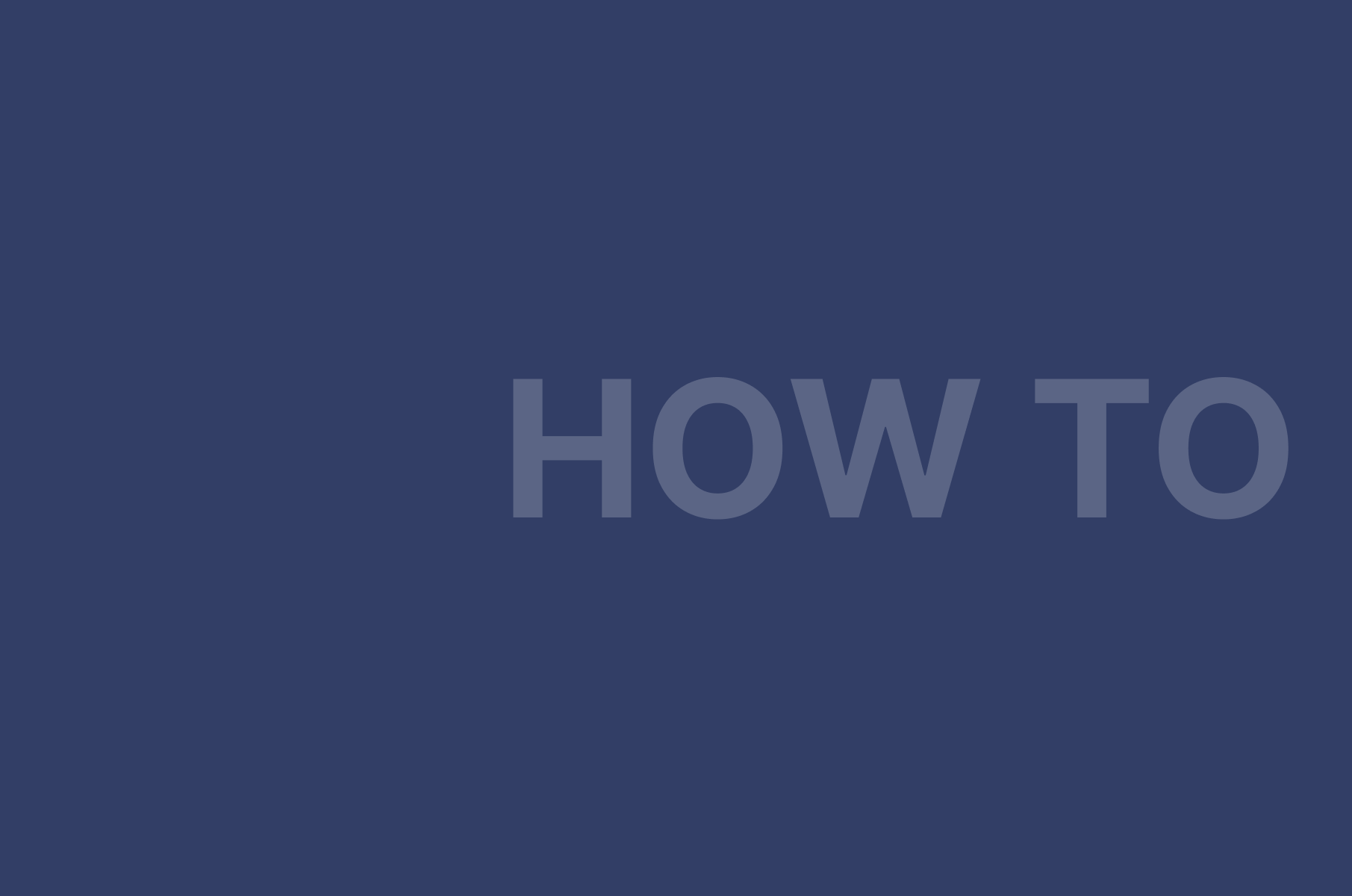
How to Configure Your Team Settings with the Digital Samba Developer API
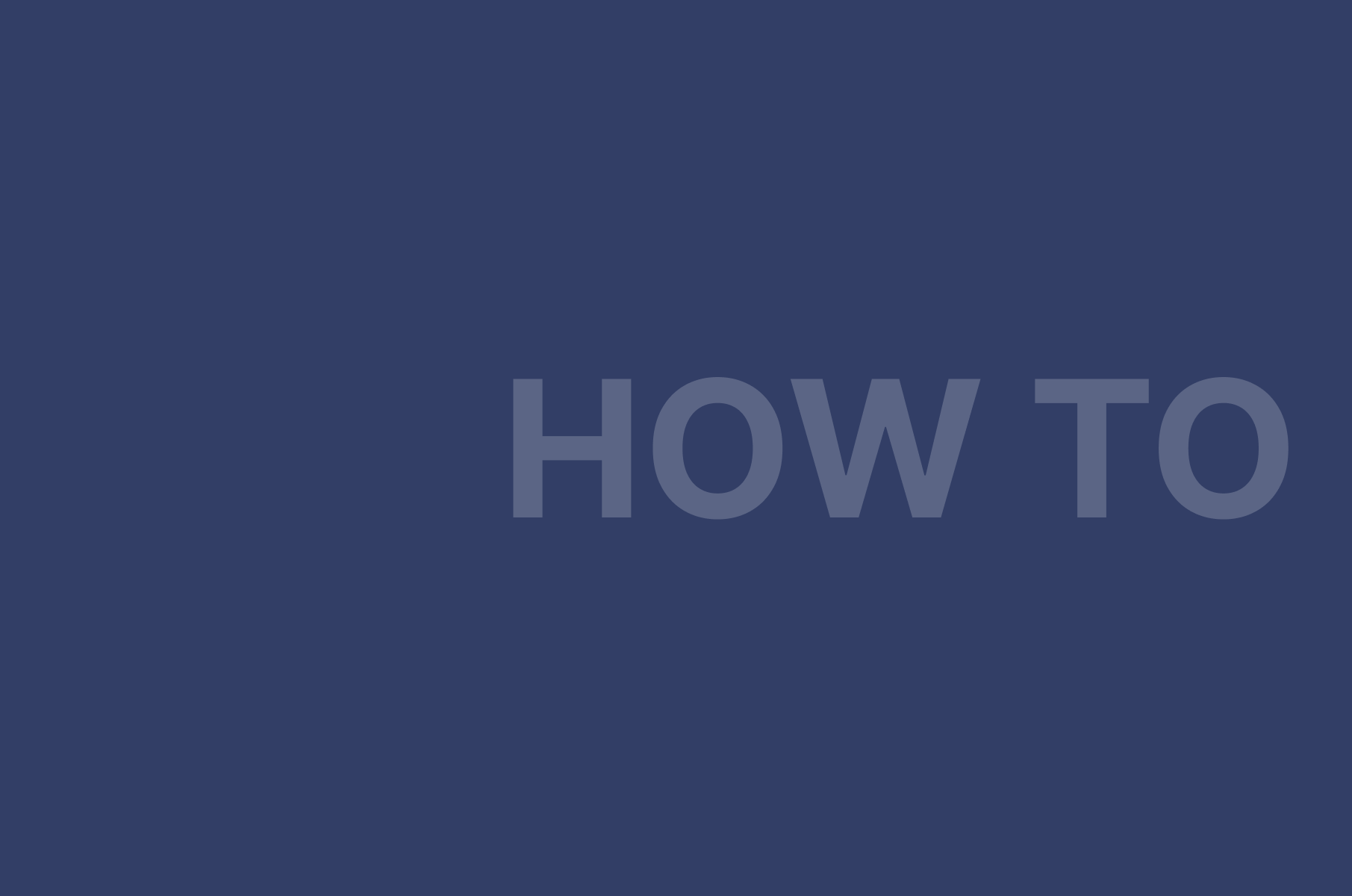
How to configure your Digital Samba Rooms using developer API and SDK
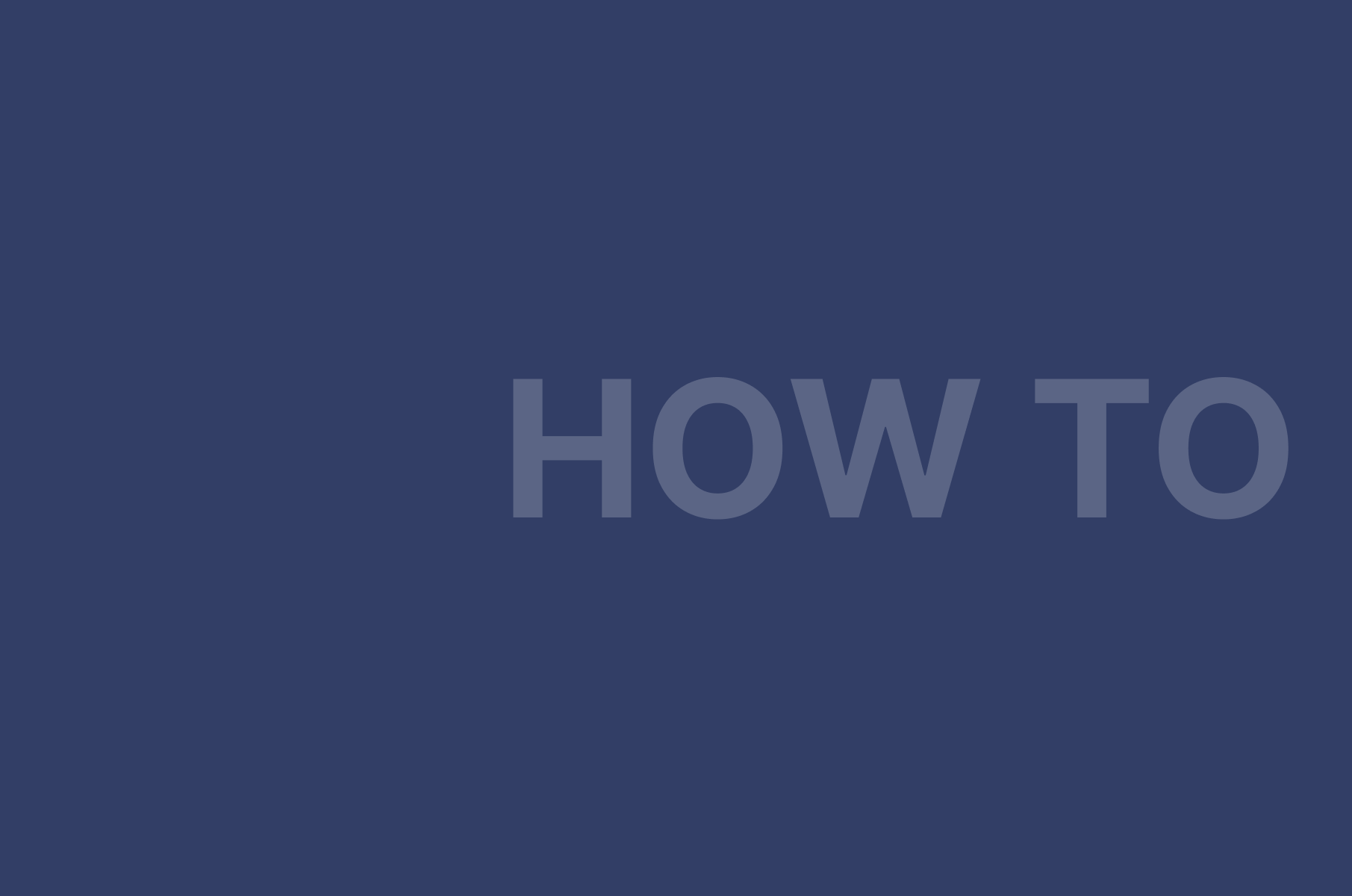